Read Each Line in a File in Python
What is Python readline?
Python readline() is a file method that helps to read one complete line from the given file. It has a trailing newline ("\n") at the end of the string returned.
You tin also make use of the size parameter to get a specific length of the line. The size parameter is optional, and past default, the unabridged line will be returned.
The flow of readline() is well understood in the screenshot shown below:
You have a file demo.txt, and when readline() is used, it returns the very first line from demo.txt.
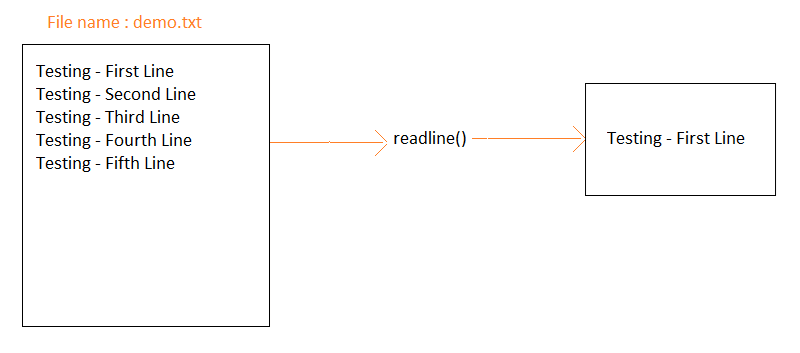
In this tutorial, yous will learn:
- Python File readline
- Characteristic of Python readline()
- Syntax
- Instance: To read the kickoff line using readline()
- Example: Using size argument in readline()
- Basic File IO in Python
- Read a File Line-by-Line in Python
- How to read all lines in a file at one time?
- How to read a File line-by-line using for loop?
- How to read a File line-by-line using a while loop?
Feature of Python readline()
Here, are of import characteristics of Python read line:
- Python readline() method reads just one complete line from the file given.
- It appends a newline ("\north") at the finish of the line.
- If you open the file in normal read mode, readline() will return you the string.
- If you open the file in binary style, readline() will return yous binary object.
- You tin can give size every bit an argument to readline(), and it volition get yous the line as per the size given inclusive of the newline. By default, the size is 0, and it returns the unabridged line.
Syntax
file.readline(size)
Parameters
size: (optional) Here, you can specify the number, an integer value to readline(). It volition get the string of that size. By default, the value of size is -i, and hence the entire string is returned.
ReturnValue
The readline() method returns the line from the file given.
Instance: To read the first line using readline()
Hither volition understand how to read the line from the file given using the readline() method. We are going to make use of demo.txt file here to read the contents.
The file contents of demo.txt are as follows:
demo.txt
Testing - FirstLine Testing - SecondLine Testing - 3rd Line Testing - Fourth Line Testing - Fifth Line
The following are the steps to read a line from the file demo.txt.
Step 1)
First, open the file using the file open() method, as shown below:
myfile = open("demo.txt", "r")
The open() method takes the first parameter as the proper noun of the file, and the second parameter is the way is while y'all want to open. Correct at present, we have used "r", which means the file will open in read mode.
Step 2)
Apply the readline() method to read the line from the file demo.txt as shown below:
myline = myfile.readline()
Step 3)
The line read is stored inside myline. Let us now print the line to see the details:
print(myline)
Pace 4)
Once the reading is washed, shut the file using shut() method as shown below:
myfile.close()
The entire code is every bit follows:
myfile = open up("demo.txt", "r") myline = myfile.readline() print(myline) myfile.close()
Output:
Testing - FirstLine
Case: Using size argument in readline()
We take seen how to read the entire line from the file given. You lot can also make use of the size parameter to get but the required length of the line.
The given example has the size parameter given as 10. The commencement line will be fetched, and it will render the line with characters from 0 to 10.
Nosotros are going to make apply of demo.txt file used earlier. Relieve the file demo.txt and use the location of the demo.txt inside open() office.
myfile = open up("demo.txt", "r") myline = myfile.readline(10) print(myline) myfile.shut()
Output:
Testing -
Basic File IO in Python
The basic file IO in Python to open a file for reading or writing is the congenital-in open() function. The two of import arguments that goes in open() role are the file path, which is a string, and the mode that specifies whether the file is meant for reading or writing. The mode statement is a cord.
Syntax:
open("file path", "mode")
Following are the modes available that tin be used with open up() method:
Mode | Clarification |
---|---|
R | This will open() the file in read way. |
West | Using w, you can write to the file. |
a | Using a with open up() volition open the file in write mode, and the contents will be appended at the end. |
rb | The rb mode volition open the file for binary data reading. |
wb | The wb mode will open the file for writing binary data. |
Since we need the file for reading, nosotros are going to make use of read mode i.eastward. (r).
Read a File Line-by-Line in Python
The readline() method helps to read just one line at a time, and information technology returns the first line from the file given.
Here, nosotros will make utilize of readline() to read all the lines from the file given. The file that will read is demo.txt. The contents of the file are:
Relieve the file demo.txt and employ the location of demo.txt inside open() function.
Testing - FirstLine Testing - SecondLine Testing - 3rd Line Testing - Quaternary Line Testing - 5th Line
Using readline() inside while-loop will take care of reading all the lines nowadays in the file demo.txt.
myfile = open("demo.txt", "r") myline = myfile.readline() while myline: impress(myline) myline = myfile.readline() myfile.close()
Output:
Testing - FirstLine Testing - SecondLine Testing - Third Line Testing - Fourth Line Testing - Fifth Line
How to read all lines in a file at one time?
To read all the lines from a given file, you can make use of Python readlines() function. The specialty of Python readlines() part is to read all the contents from the given file and save the output in a listing.
The readlines() part reads until the End of the file, making use of readline() function internally and returns a list with all the lines read from the file.
Hither is a working example to read all the lines from the file using readlines().
The file that nosotros are going to make employ of to read is test.txt. The contents of the file examination.txt are as follows:
test.txt: Save the file test.txt and utilize the location of test.txt inside open up() office.
Line No 1 Line No 2 Line No 3 Line No four Line No 5
myfile = open("test.txt", "r") mylist = myfile.readlines() print(mylist) myfile.close()
Output:
['Line No 1\n', 'Line No 2\north', 'Line No iii\n', 'Line No 4\n', 'Line No 5']
How to read a File line-by-line using for loop?
Following are the steps to read a line-by-line from a given file using for-loop:
Step1 :
First, open the file using Python open() office in read mode.
Step 2:
The open up() function will return a file handler. Use the file handler inside your for-loop and read all the lines from the given file line-past-line.
Stride 3:
Once washed, close the file handler using the close() function.
Hither is a working example of using for-loop to read line-by-line from a given file. The file that we are going to apply here is examination.txt.
The contents of test.txt are every bit shown beneath. Save the file test.txt and employ the location of test.txt within an open() office.
Line No 1 Line No 2 Line No 3 Line No 4 Line No five
myfile = open("examination.txt", "r") for line in myfile: print(line) myfile.close()
Output:
Line No 1 Line No 2 Line No 3 Line No four Line No 5
How to read a File line-by-line using a while loop?
You tin can make use of a while loop and read the contents from the given file line-past-line. To do that, commencement, open the file in read way using open() function. The file handler returned from open(), use it inside while –loop to read the lines.
Python readline() function is used inside while-loop to read the lines. In the case of for-loop, the loop terminates when the end of the file is encountered. Simply the same is not the case with a while-loop, and you lot need to keep a check to see if the file is washed reading. So one time the readline() function returns an empty string, you tin can make utilise of the break statement to cease from the while –loop.
Here is a working example to read a file line by line using a while-loop.
The file that nosotros are going to make use is examination.txt .Save the file test.txt and use the location of exam.txt inside open() function.
Line No 1 Line No 2 Line No iii Line No 4 Line No v
myfile = open("test.txt", "r") while myfile: line = myfile.readline() print(line) if line == "": break myfile.close()
Output:
Line No 1 Line No 2 Line No 3 Line No iv Line No 5
Summary
- Python readline() is a file method that helps to read i consummate line from the given file. Information technology has a trailing newline ("\n") at the end of the string returned.
- You can also make apply of the size parameter to get a specific length of the line. The size parameter is optional, and by default, the entire line volition be returned.
- The readline() method helps to read just one line at a time, and it returns the first line from the file given. We will make utilize of readline() to read all the lines from the file given.
- To read all the lines from a given file, you can make use of Python readlines() function. The specialty of Python readlines() part is that information technology reads all the contents from the given file and saves the output in a list.
- The readlines() role reads till the End of the file making use of readline() function internally and returns a list that has all the lines read from the file.
- It is possible to read a file line by line using for loop. To exercise that, first, open up the file using Python open() function in read style. The open() office will return a file handler. Utilize the file handler inside your for-loop and read all the lines from the given file line by line. Once done,shut the file handler using close() function.
- You can make use of a while loop and read the contents from the given file line by line. To practise that, kickoff, open the file in read way using open up() office. The file handler returned from open(), use it within while –loop to read the lines. Python readline() office is used inside while-loop to read the lines.
Read Each Line in a File in Python
Source: https://www.guru99.com/python-file-readline.html
0 Response to "Read Each Line in a File in Python"
Post a Comment